What is the sum of all odd numbers in the Fibonnoci sequence that are less than 5000?
class Fibonacci
def initialize
@a = 1
@b = 1
@total = 0
end
# Example #1 uses @instance variables
# of a class
def sum_odd(number)
while @a <= number
if @a.odd? # Syntactic Sugar
@total += @a
end
@a, @b = @b, @a + @b
end
puts %Q{ Total: #{@total} }
end
# Example #2 uses local variables.
# I wrote this to make it easy
# to re-write in Javascript or Python
def sum_even(number)
a = 1
b = 1
total = 0
while a <= number
if a % 2 == 0
total += a
puts %Q{ Is Even Number }
else
puts %Q{ Is Odd Number }
end
a, b = b, a + b
end
puts %Q{ Total: #{total} }
end
end
fib = Fibonacci.new
fib.sum_odd(5000)
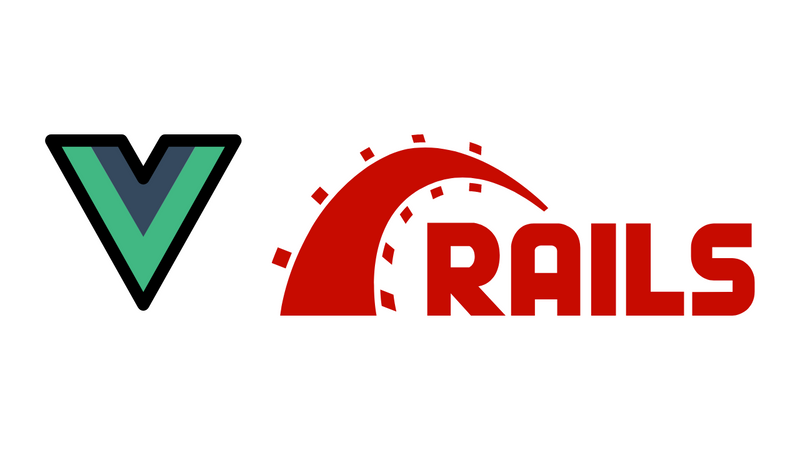
Deploying Rails 5.x on AWS ElasticBeanstalk using AWS CodeCommit
How to deploy your Rails app on ElasticBeanstalk (including S3 buckets, security groups, load balancers, auto-scalling groups and more) using CodeCommit.

Subscribe to new posts
Processing your application
Please check your inbox and click the link to confirm your subscription
There was an error sending the email