
Swift
17 postsMy journey through Apple Swift, a new programming language for iOS, OS X, watchOS, and tvOS apps.



Swift: Do Try Catch
I've discovered that there are really two ways to accomplish a Try/Catch strategy in Swift. The first example is technically correct but the "shorthand&...
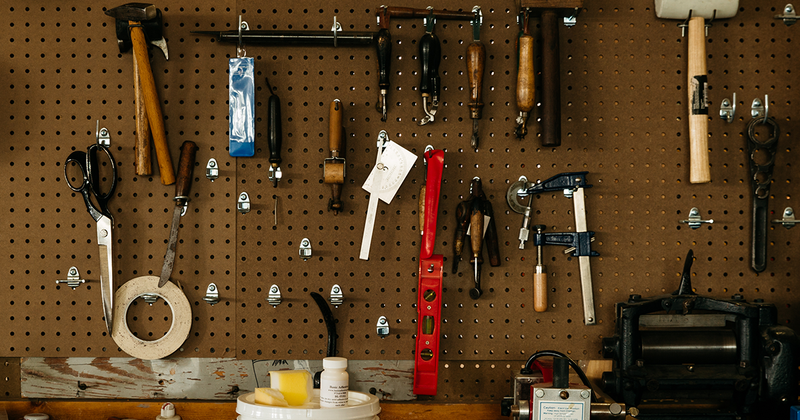

Swift: Load a Wav sound using AVFoundation
I sometimes need to dynamically load a .wav file. Here's how I do it using AVFoundation. func changeSound(_ song:(path: String, format: String)){ var err:NSError?...
Swift: Get Random Boolean
This function will help you get a random true or false boolean value. func randomBool() -> Bool { return arc4random_uniform(2) == 0 ? true : false }...
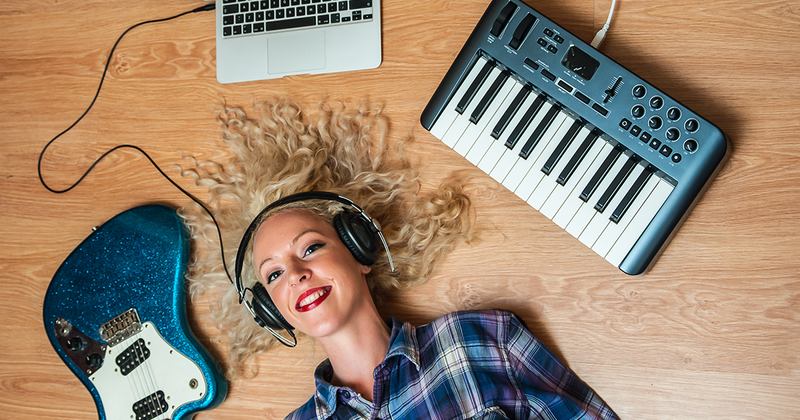
Curated list of audio frameworks and libraries for iPhone and OS X
A curated list of awesome audio frameworks, libraries, and software for iOS, Mac OSX and tvOS. Audio Production * AudioKit for iOS and OS X [https://github.com/audiokit/...
Alcatraz the package manager for xCode
As a full-stack developer, I find myself using more and more package managers every year. For example, here's my current set-up: * homebrew or apt-get for downloading...

Learning Swift Lang
I first started writing HTML + Javascript when I was 17 years old. No one told me that I should be a coder nor did anyone bother to tell...
Decompiling your iPhone app
If you ever end up losing your source code and you have no other option than decompile your iPhone app, here are a list of tools: * Class Dump...

Swift: Simple GET Request
A simple GET request using Swift. //: Playground - noun: a place where people can play import UIKit class Test { init(path:String){ getRequest(path) } func getRequest(path:String)...
Common Swift string extensions
String Extension import Foundation extension String { var length: Int { get { return countElements(self) } } func contains(s: String) -> Bool { return self.rangeOfString(s) ? true : false } func replace(...